Diving into Sui Bridge Architecture
Sui Bridge is a native bridge for Sui offering a trust-minimized avenue to bridge assets to and from Sui.
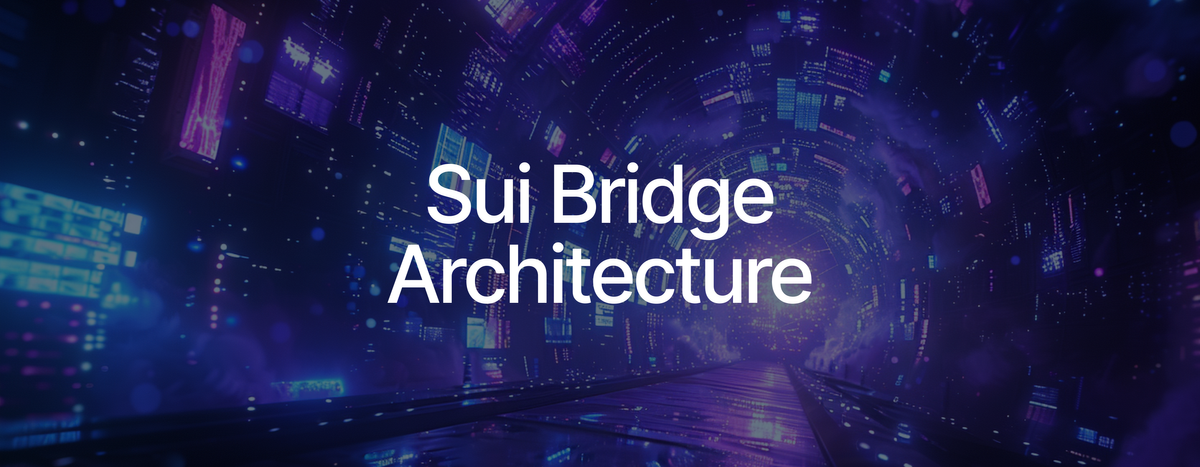
Over the past few months, significant efforts have been dedicated to developing Sui Bridge, a native bridge for the Sui ecosystem. This initiative enhances the accessibility of Sui for the broader community and improves interoperability with other blockchains.
Sui Bridge, a critical component in the Sui ecosystem, facilitates the secure and efficient transfer of assets and data between Sui and other blockchain networks. This capability not only broadens reach for apps built on Sui but also offers an avenue to integrate more deeply into the wider blockchain landscape. Interoperability increases growth and adoption of Sui, enabling assets on other networks, such as Ethereum, to safely and easily migrate to Sui.
Sui Bridge background
In the context of the blockchain ecosystem, a bridge is a protocol that enables interaction and communication between independent blockchain networks. Bridges facilitate the transfer of data across the boundaries, allowing users to move their assets from one chain to another and even do more complex operations such as cross-chain message passing.
Amongst the handful of bridge design choices, Sui Bridge utilizes a lock and mint mechanism, one of the most widely used solutions. As a lock and mint design, Sui Bridge holds Ethereum-native assets within Ethereum smart contracts, whereas it mints or burns Sui asset depending on the direction of the bridge interaction.
As a native bridge on Sui, Sui Bridge requires no additional trust. The entities that secure the Sui are the same that secure Sui Bridge and the code for Sui Bridge is baked into the Sui framework.
In its current state, Sui Bridge is available on Testnet and supports bridging of tokens such as ETH, WETH, WBTC, and USDT between Ethereum Sepolia and Sui Testnet. After Sui Bridge is released on Mainnet, support for more assets will be prioritized. Later versions of Sui Bridge will add new functionality such as custom cross-chain messaging and integration with other blockchains.
High level architecture
Sui Bridge has four key components: the Sui Bridge committee or node network, the Sui Bridge smart contracts, the Full nodes operating on Ethereum and Sui, and the bridge client.
The client is the interface between the user and the Sui Bridge infrastructure. It coordinates user bridging actions by submitting correctly formatted transactions and collecting Sui Bridge node signatures as needed. The client uses full nodes to submit transactions on both sides of the bridge. The Sui Bridge nodes also run Ethereum and Sui full nodes to listen for bridging actions and respond to them through the following transaction. While the bridge client is permissionless and can be executed by anyone, many bridge nodes will toggle on bridge clients to ensure the liveness of the network.
When bridging assets from Ethereum to Sui, the user deposits assets to the Sui Bridge smart contract on Ethereum. The client then observes this transaction and coordinates the bridging process. The Sui Bridge committee operates Ethereum full nodes that also listen for such bridging actions to verify that what the client is requesting is legitimate.
After verification, the bridging action is completed by minting of bridged assets for the user through the Sui Bridge package on Sui. With such low costs to bridge, Sui Validators currently subsidize the gas fees associated with bridging transactions on Sui and allowing the client to execute the transactions automatically, creating a seamless bridging experience onto Sui.
When bridging from Sui to Ethereum, the process is similar except users must submit the claiming transaction manually on Ethereum. This transaction includes signature data from the Sui Bridge nodes in the transaction calldata that allows the Ethereum account to redeem the specified assets locked in the bridge contract.
Additionally, all bridging records and approvals are stored in the bridge object onchain. This is feasible in Sui because its storage and gas costs are affordably low. The Sui bridge contract also handles governance actions, which are controlled by the Sui Bridge committee.
Bridge messages
To ensure low gas costs, Sui Bridge constructs messages in a lightweight manner that is easy for the receiving chain to decode. A common messaging format is employed to ensure that each chain can efficiently decode and verify messages and signatures.
Move code example:
public struct BridgeMessage has copy, drop, store {
message_type: u8,
message_version: u8,
seq_num: u64,
source_chain: u8,
payload: vector<u8>
}
Solidity code example:
struct Message {
uint8 messageType;
uint8 version;
uint64 nonce;
uint8 chainID;
bytes payload;
}
These bridge messages are designed with simplicity and efficiency in mind. The structure is minimalistic, containing only essential fields such as message type, version, sequence number, source chain identifier, and payload. This streamlined design reduces complexity and computational overhead, ensuring low gas costs while facilitating fast and reliable cross-chain communication.
Bridge security
Determining a bridge’s trust model is one of the most important design decisions when developing a bridge to support a bustling ecosystem and large flows. A bridge should be both secure and decentralized. While in some bridge designs these attributes may be at odds with each other, developing a native bridge offers the opportunity to leverage the security of Sui to secure Sui Bridge.
The same node operators that secure Sui by running validator nodes manage and maintain the infrastructure that the Sui Bridge runs on. Sui Bridge inherits a decentralized network of node operators that are highly capable of running and securing Sui infrastructure.
As mentioned above, most Sui Bridge operations occur on Sui and treat Sui as a control panel for the bridge. This benefits from the security that software developed in Move inherits.
Bridge Committee
Sui Bridge is safeguarded by the same set of validations that secures Sui. During the Testnet phase, the committee consists of a subset of Testnet validators. When on Mainnet, the majority if not all active Sui validators will be part of the Bridge Committee. Dynamic management of the committee will be implemented post-Mainnet to enable newer validators joining. Allowing only Sui validators to be a part of the Sui Bridge Committee ensures that the security assumptions, properties, and social consensus are inherited.
To maintain high security and compatibility with other blockchain networks, the Sui Bridge employs the Elliptic Curve Digital Signature Algorithm (ECDSA) for the Bridge Committee signatures. By leveraging ECDSA, Sui Bridge ensures seamless interoperability and secure transaction verification, reinforcing the integrity and trustworthiness of the system.
Signature verification
Sui Bridge utilizes recoverable ECDSA signatures, which allow public key recovery directly from the signature itself. This feature streamlines the verification process by enabling us to retrieve the public key and confirm the authenticity and integrity of the signature without requiring prior knowledge of the public key.
A message is considered valid only when the combined weight of the signatures meets or exceeds a predefined threshold. This threshold mechanism ensures that a sufficient number of authenticated signatures are required to validate a message, thereby enhancing the security and reliability of the system. By implementing this approach, we safeguard against fraudulent activities and ensure that only legitimate transactions are processed.
Code example in Move:
...
let mut message_bytes = SUI_MESSAGE_PREFIX;
message_bytes.append(message.serialize_message());
let mut threshold = 0;
while (i < signature_counts) {
let pubkey = ecdsa_k1::secp256k1_ecrecover(&signatures[i], &message_bytes, 0);
// check duplicate
// and make sure pub key is part of the committee
assert!(!seen_pub_key.contains(&pubkey), EDuplicatedSignature);
assert!(self.members.contains(&pubkey), EInvalidSignature);
// get committee signature weight and check pubkey is part of the committee
let member = &self.members[&pubkey];
if (!member.blocklisted) {
threshold = threshold + member.voting_power;
};
seen_pub_key.insert(pubkey);
i = i + 1;
};
...
Code example in Solidity:
function verifySignatures(bytes[] memory signatures, BridgeUtils.Message memory message)
external
view
override
{
uint32 requiredStake = BridgeUtils.requiredStake(message);
uint16 approvalStake;
address signer;
uint256 bitmap;
// Check validity of each signature and aggregate the approval stake
for (uint16 i; i < signatures.length; i++) {
bytes memory signature = signatures[i];
// recover the signer from the signature
(bytes32 r, bytes32 s, uint8 v) = splitSignature(signature);
(signer,,) = ECDSA.tryRecover(BridgeUtils.computeHash(message), v, r, s);
require(!blocklist[signer], "BridgeCommittee: Signer is blocklisted");
require(committeeStake[signer] > 0, "BridgeCommittee: Signer has no stake");
uint8 index = committeeIndex[signer];
uint256 mask = 1 << index;
require(bitmap & mask == 0, "BridgeCommittee: Duplicate signature provided");
bitmap |= mask;
approvalStake += committeeStake[signer];
}
require(approvalStake >= requiredStake, "BridgeCommittee: Insufficient stake amount");
}
Building interoperability
The Sui native bridge not only provides a secure and efficient means of transferring assets between blockchain networks but also lays the groundwork for more advanced cross-chain interactions. By leveraging its robust trust model, incorporating ECDSA for secure and verifiable transactions, and employing a committee-based signature verification process, Sui Bridge ensures high security and reliability while still remaining flexible.
The scalability and flexibility of the Sui bridge architecture allow for future expansions and integrations with other blockchain networks. As the ecosystem develops, the bridge will support a wider array of assets and abilities, such as custom cross-chain messaging allowing for unique cross-chain interactions.
Sui Bridge represents a significant step forward in the landscape of Sui interoperability, offering a seamless and highly secure solution for cross-chain asset transfers. As Sui Bridge launches on Mainnet, users can look forward to an increasingly robust and versatile bridge that meets current demands while also anticipating future needs.